Proxy Pattern
When use the Proxy Pattern
● A remote proxy provides a local representative for an object ina different address space.
● A virtual proxy creates expensive objects on demand.
● A protection proxy controls access to the original object.
Protection proxies are useful when objects should have different
access rights.
Example - Virtual Proxy Example.
Consider an image viewer program that lists and displays high resolution photos. The program has to show a list of all photos however it does not need to display the actual photo until the user selects an image item from a list.
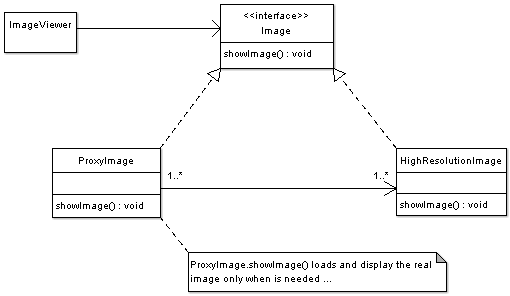
Step 1
Create an interface.
Image.java
public interface Image { void display(); }
Step 2
Create concrete classes implementing the same interface.
RealImage.java
public class RealImage implements Image { private String fileName; public RealImage(String fileName){ this.fileName = fileName; loadFromDisk(fileName); } @Override public void display() { System.out.println("Displaying " + fileName); } private void loadFromDisk(String fileName){ System.out.println("Loading " + fileName); } }
ProxyImage.java
public class ProxyImage implements Image{ private RealImage realImage; private String fileName; public ProxyImage(String fileName){ this.fileName = fileName; } @Override public void display() { if(realImage == null){ realImage = new RealImage(fileName); } realImage.display(); } }